According to the official React Native docs, their AsyncStorage module has been deprecated. Instead, they recommend using one of the community packages. This is why, in this guide, we have chosen to cover one of these:
@react-native-async-storage/async-storage
What does this package do?
Its formal definition states that this library is an asynchronous, unencrypted, persistent, key-value storage API.
Well, those are a lot of adjectives for a storage API. Let’s break this terminology down. Asynchronous means that each of its methods returns an object that could be a Promise or an Error. It’s unencrypted, so beware of using it to store sensitive data. Being persistent means it saves the data, even when the user has closed the API or even the device. Finally, key-value pairs are the way data is stored.
Now that we know what it is, let’s see how useful it is.
Why should you use it?
First of all, it’s a lightweight solution that doesn’t involve a database, server or even an internet connection. It sounds like a good choice, but remember it is just one more alternative for storing information and sharing data in your application; it may not always be the best one to use.
So the really important question would be when to use this library, rather than why you should use it. Based on the characteristics we mentioned, you can deduce when it is appropriate to use AsyncStorage and when it is not such a good idea.
It is usually replaced by React Context or Redux, but despite being used for the same purpose, they are not the same. Mainly because React Context and Redux store data in memory that is erased when the app closes and AsyncStorage saves data persistently in the device. It resembles the local storage used for web applications.
How to use it
There are three main actions you can take with AsyncStorage. Set a key-value pair, get a value of a given key and remove the pair using its key. The API methods used for this are setItem, getItem and removeItem, respectively. You can delve into the API if you are interested, but we will only use these main ones in the following example.
Example of React Native AsyncStorage
Let’s do a proof of concept. Our application will store the user’s theme preference, whether light or dark. For this purpose, we are not compromising data scalability, sensitive information or anything else we may be worried about.
Start by installing create-react-native-app globally with yarn by running this command:
yarn global add create-react-native-app
Now, you can run create-react-app asyncstorapp to create your React Native application. We decided to use “asyncstorapp”, but you can name it however you want. Follow the instructions and you are done. In our case, the project was created with react-native version 0.63.4.
Proceed to install the package by running:
yarn add @react-native-async-storage/async-storage
We will be using the 1.15.5 version. According to the official installation guide, running npx pod-install is needed to link this dependency.
You can now enter npm start in your terminal and use a real or virtual device to start testing what we are about to develop.
Let’s import it and try it out. As a first approach, we will store a hardcoded key-value pair, then close the app and open it again to prove it’s being saved.
To start, add the following to your App.js file and then look at the console.
Link: script
Remember it is asynchronous, so that’s why try/catch and async/await are used to handle the Promise in getItem.
As you can see, value null has been outputted. That’s because we haven’t set a value for that key yet. So let’s create an asynchronous setItem function that does it.
Also, let’s change our code so instead of getting the theme value in the useEffect, we store it.
Keep in mind that AsyncStorage can only store string values. In order to store object data you need to serialize it first. You can do this by using JSON.stringify() to store it and JSON.parse() to retrieve it.
In this case, we will be using just a string, so it should look like this:
Link: script
To test if the dark value has been stored correctly, change the useEffect again to its previous value. Save your file and you will see value dark outputted in your terminal.
Let’s close and reopen the application to test if that value has been saved to our device. You will see the same value in your terminal. This proves it’s really working.
Now that we know it works, it’s time to store and use our application theme properly. Replace your code with the following:
Link: script
You will see something like this, working like a light switch, lighting up when turned on and darkening when turned off:
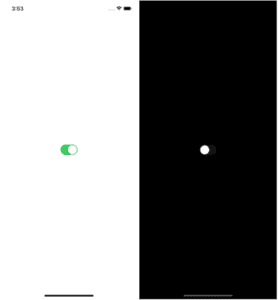
And that’s it! Close the app keeping in mind the previous state and reopen it so you can confirm that it remembers it.
Final thoughts on React Native AsyncStorage
This package fulfills a need when you wish to recover data without depending on anything other than your device. However, it also has some drawbacks.
Since it is a storage option that is on your device until it is manually removed, we find it very useful as long as the data you are storing or sharing in your application has been carefully selected. When it comes to saving sensitive data or large amounts of it, we would recommend choosing another alternative.