In this guide, we will cover how to connect React Native and Node.js to develop a login application. Once logged in, users will be able to access a private resource. We won’t go into too much detail, so you can get started as soon as possible.
Before we start, let’s take a look at why we chose to use these technologies. You should know that prior knowledge of both tools is required to complete this guide successfully.
Why React Native?
According to the official docs, “React Native combines the best parts of native development with React, a best-in-class JavaScript library for building user interfaces.”
To put it more simply, React Native is the adaptation of React for native development. But let’s analyze what that really means.
React Native is a framework based on React that uses JavaScript to build mobile applications that render natively, for Android and iOS at the same time.
Therefore, this tool allows web developers to take advantage of React’s outstanding benefits and apply their prior JavaScript knowledge but, in this case, to target mobile platforms.
Why Node.js?
The official documentation claims that “Node.js is a JavaScript runtime built with Chrome’s V8 JavaScript engine.”
Being a runtime means it acts as an environment that allows you to execute, in this case, a JavaScript program on your machine. Moreover, it uses a non-blocking I/O model to respond to events from a user or from another application, which makes it lightweight and efficient.
Moreover, its package ecosystem, npm, has the greatest number of open-source libraries in the world.
Now that we know which technologies we are going to use, let’s get started.
Create the Node.js project
Let’s talk about our backend and what its role will be. It will receive requests from the frontend and, in turn, give a response according to the result of the data flow. Behind the scenes, it will be interacting with the database, creating the users when they sign up and verifying their credentials when they attempt to log in.
Requirements
To be able to follow this part of the guide, you must have Node installed. We will be using node v14.16.1 and the npm 6.14.12 version.
To store our users in the database, we will be using MySQL Community Server as well as MySQL Workbench, so you will need them both as well. You can use another database if you want. If you do, you can skip the next step.
How to setup MySQL 
Start by downloading MySQL Community Server from their website. Follow the installation instructions and that’s it. Then, MySQL Workbench will be needed to manage our database. Download it from the same site and install it. We will use versions 8.0.25 and 8.0.12, respectively.
When you have finished, you will see a MySQL local instance running. To connect to the database, click on that instance and you will be asked to enter a password. Remember the password, as well as the username and the port your local instance is running on. You will need that later.
Now, it’s time to create our database. Right click below “Schemas” and choose “Create schema”. We will name it “loginDB”, but you can choose whichever name you prefer. Click “Apply” and the database will be created.
In the left panel, you will see the database we have just created. Inside it, there is a “Tables” section. Right click it and select “Create table”. Select the configuration below and click “Apply” to finish the setup.
Once you are done, you can start by creating the backend project and installing the necessary dependencies.
First, we will create a “loginApp” folder and, inside it, another one called “server” where our backend will be placed. Folder names are up to you, you can name them however you want. Then, run the npm init command to initialize npm and proceed to install the required dependencies listed below. To install them, we will be using yarn version 1.22.10 and running the yarn add <package_name> command. we will summarize what each dependency does below, in case you are not familiar with all of them.
Package | Version | Description |
bcryptjs | 2.4.3 | Hashes and compares user passwords |
express | 4.17.1 | Minimal and flexible framework to be used |
jsonwebtoken | 8.5.1 | Generates and verifies request tokens |
mysql2 | 2.2.5 | Allows you to interact with the MySQL database |
nodemon | 2.0.7 | Automatically restarts the application when file changes in the directory are detected |
sequelize | 6.6.2 | ORM to be used |
After installing them, go to your package.json file and, in the scripts section, change start from node app.js to nodemon app.js. You can now run npm start and nodemon will be activated.
Enough with the preparations, let’s code. In the “server” folder, create an app.js file that will initialize the application and create and hold the environment configuration. Place this in your file:
app.js
Link: script
From now on, pay close attention to the imports in each file to understand how they are connected.
As you may see, in the first line of our app.js, we are importing express and, at the bottom, we are specifying the port where our server will be listening for requests. Also, two files we haven’t talked about yet are being imported. app.js will connect what they provide to our application.
The first one is database.js and it is inside a “utils” folder. Here, we will create an instance of sequelize that will let us connect to our MySQL database. Copy the following content and replace your password where it says YOUR_PASSWORD.
database.js
Link: script
The second imported script is inside a “routes” folder and is named routes.js. This is the one that defines our API endpoints using express, as follows:
routes.js
Link: script
We are only going to use the first three endpoints, the other two are examples you may need to define a public route and a default one.
You may note that, in this script, another file came into play in the imports. Its name is auth.js and it is placed inside the “controllers” folder. This file contains the actions that get executed when those requests are received.
So let’s see what auth.js should include:
auth.js
Link: script
Again, looking at the imports, we can see that there are two new packages: bcryptjs and jsonwebtoken.
There is also another file we haven’t seen yet. Let’s look at what it should include before continuing with auth.js.
The file name is user.js and it is inside a “models” folder. It should import the sequelize instance from the database.js file we already saw and, then, define the users table we are going to store. Copying this into your file will be enough:s
user.js
Link: script
Going back to the auth.js file, we can see three functions are being exported: signup, login and isAuth. These will be in charge of resolving the requests defined in routes.js.
signup registers users in the database. First, it checks if the email provided has already been registered. Then, if the email and password have been received, it hashes the password and stores the user in the database.
login handles login requests. It starts by checking if the email corresponds to a database user. If that’s the case, it hashes the password and compares it to the user password in the database. If they match, it responds with a temporary secret token.
isAuth asks for the secret token and, if it is provided, proceeds to verify it. If everything goes well, it will finally respond with the private resource. In this case, that resource will be just a message containing “here is your resource”. This is enough for our proof of concept.
That’s it. We are done for now. We have our server ready to respond to authentication requests in port 5000 after running npm start. You can test the endpoints defined using Postman or another tool of your choice.
Create the React Native project
It’s time to talk about the frontend and what its role in this project should be. It will receive the user input and send a request to the backend, to sign up or log in, depending on what the user has selected. Then, based on the backend response, it will show an error or success message to the user.
So let’s start coding. The create-react-native-app package will set up the project for you. First, run the following command to install it globally: yarn global add create-react-native-app
Then, go to your “loginApp” folder and execute the command we’ve just installed by running create-react-native-app mobile. Again, we decided to use “mobile” as the name of the frontend app, but it’s up to you.
This step is optional since your app’s style is up to you. Inside your new “mobile” folder, create a new folder called “public” and another one named “images” inside it. That last folder will contain the background image for the app. We downloaded the one we will be using from here and we had to rotate it to achieve a portrait orientation.
Then, create another folder inside “mobile” called “screens”. Inside it, create two files, “index.js” and “AuthScreen.js”. The first one will be the one that exports the screen like this:
index.js
Link: script
The second one will contain our unique screen, where the user can get authenticated. It will use fetch to send the user requests to the backend and return a message according to what it receives. There will be one request sending user input and creating the signup or login, and another one requesting the private resource. Once the user logs in, the resource request will automatically be triggered.
We will be using a single screen for the entire app to make it simpler, but you can try a different approach if you want. Copy its content to your AuthScreen.js file:
AuthScreen.js
Link: script
Finally, we need to import that screen to our App.js file and render it as follows:
App.js
Link: script
And that’s all. Just run npm start on the mobile directory and the frontend app will start running on port 3000. Then, proceed to use a virtual or real device to test how it communicates with the backend. You should see something like this:
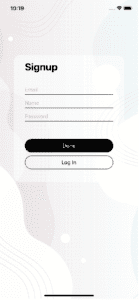
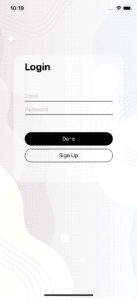
Let’s test if it works:
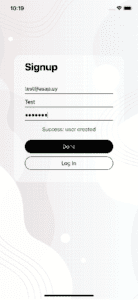
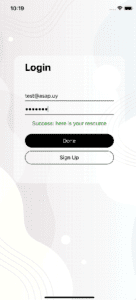
Let’s test error messages by creating a user with the same email and trying to log in with the wrong credentials:
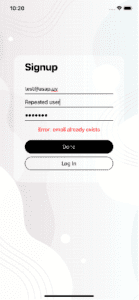
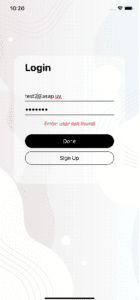
It works perfectly! After you finish this guide, your directory should look like this:
You have reached the end of this guide. Now you have a React Native frontend login application working with a Node.js backend. Congratulations!
Challenge: Develop tests for your React Native App
We encourage you to test your application on both sides to ensure it is working as expected. Testing is an essential part of any development process and you should start incorporating it into your projects from the beginning.
You can start with the frontend side, testing components in the way a user would use them. We recommend using React Testing Library. If you haven’t heard about it, you can read the article we have written about it.
Final thoughts on React Native with Node.js backend
To sum up, both React Native and Node.js are powerful tools that share a single programming language: JavaScript. With their separate advantages combined, you have built an incredibly strong mobile application. We have proven how straightforward it is to get started with any of them, even if you have not seen it before. We hope this guide gives you a starting point to start creating mobile apps with more functionalities.